8.12
3 Pict Constructors🔗ℹ
Creates a blank
static pict with the specified bounding box.
Creates a
pict to draw a rectangle. If an
around pict is
provided, then it both supplies default
width and
height values an is
overlayed on top of the rectangle
image,
The rectangle has an outline if line is not #false,
and it is filled in if fill is not #false. If the
rectangle has an outline, line_width is used for the outline. A
line, fill, or line_width can be
#'inherit to indicate that a context-supplied color and line
width should be used. See also Pict.colorize and
Pict.colorize Pict.line_width.
If rounded is a non-negative number, it is used as the radius
of an arc to use for the rectangle’s corners. If rounded is a
negative number, it is negated and multipled by the rectangle’s width
and height to get a radius (in each direction) for the rounded corner.
When the refocus_on argument is a pict, then
Pict.refocus is used on the resulting pict with
refocus_on as the second argument. If refocus is
#'around and around is not #false, then the
pict is refocused on around, and then padded if necessary to
make the width and height match width and height.
The epoch_align and duration_align arguments are
used only when around is supplied, and they are passed on to
overlay to combine a static rectangle pict with
around. The order argument is similarly passed along to
overlay. If around is #false, the
resulting pict is always a static pict.
A shorthand for
rectangle where the width and height are
specified as
size.
> square(~around: text("Hello"), ~fill: "lightgreen") |

|
|
fun ellipse( | ~around: around :: maybe(Pict) = #false, | ~width: width :: Real: if around | Pict.width(around) | 32, | ~height: height :: Real: if around | Pict.height(around) | width, | ~arc: arc :: maybe(ArcDirection) = #false, | ~start: start :: Real = 0, | ~end: end :: Real = 2 * math.pi, | ~fill: fill :: maybe(ColorMode) = #false, | ~line: line :: maybe(ColorMode) = !fill && #'inherit, | ~line_width: line_width :: LineWidth = #'inherit, | ~order: order :: OverlayOrder = #'back, | ~refocus: refocus_on :: maybe(Refocus) = #'around, | ~epoch: epoch_align :: EpochAlignment = #'center, | ~duration: duration_align :: DurationAlignment = #'sustain | ) :: Pict |
|
Like
rectangle, but for an ellipse or arc/wedge. The pict
draws an arc or widge if
arc is
#'cw (clockwise) or
#'ccw (counterclockwise).
|
fun circle( | ~around: around :: maybe(Pict) = #false, | ~size: size :: Real: if around | | math.max(Pict.width(around), Pict.width(around)) | | 32, | ~arc: arc :: maybe(ArcDirection) = #false, | ~start: start :: Real = 0, | ~end: end :: Real = 2 * math.pi, | ~fill: fill :: maybe(ColorMode) = #false, | ~line: line :: maybe(ColorMode) = !fill && #'inherit, | ~line_width: line_width :: LineWidth = #'inherit, | ~order: order :: OverlayOrder = #'back, | ~refocus: refocus_on :: maybe(Refocus) = #'around, | ~epoch: epoch_align :: EpochAlignment = #'center, | ~duration: duration_align :: DurationAlignment = #'sustain | ) :: Pict |
|
> circle(~around: text("Hello"), ~fill: "lightgreen") |

|
Creates a
pict that draws a polygon. The maximum x and y values
among the
pts determine the resulting pict’s bounding box.
> polygon([[0, 0], [50, 0], [50, 50]], ~fill: "lightgreen") |
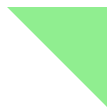
|
Creates a
pict that draws a line from the top-left of the pict.
The
dx and
dy arguments determine both the shape of
the line and the width and height of the pict.
> line(~dx: 10, ~line_width: 3) |

|
> line(~dy: 10, ~line: "blue", ~line_width: 3) |

|
> line(~dx: 10, ~dy: 10) |

|
Creates a
pict that draws text using
font> text("Hello") |

|
> text("Hi!", ~font: draw.Font(~kind: #'roman, ~size: 20, ~style: #'italic)) |

|
Creates a
pict that draws a bitmap as loaded from
path.
Creates a
pict with an arbitrary drawing context. The
draw function receives a s
draw.DC, an x-offset, and
a y-offset.
> dc(fun (dc :: draw.DC, dx, dy): | dc.line([dx, dy+10], [dx+20, dy+10]) | dc.line([dx+10, dy], [dx+10, dy+20]) | dc.ellipse([[dx, dy], [21, 21]]), | ~width: 20, | ~height: 20) |
|

|
Converts a static pict value compatible with the Racket
pict library into a
Pict value.
A color specification, where
#'inherit allows the color to be
configured externally, such as through
Pict.colorize.
A line-width specification, where
#'inherit allows the color
to be configured externally, such as through
Pict.line_width.
Refocusing options for functions like
rectangle.
Arc directions, clockwise or counterclockwise.