8.12
4 Pict Combiners🔗ℹ
Creates a pict that combines the given picts horizontally
with the first pict as leftmost.
If a pict extends horizontally outside its bounding box, then the
front-ot-back order of picts can matter for the combined image. The
order argument determines the order of each pict added to the
right of the combined image.
The picts are first made concurrent via concurrent, passing
along duration_align and epoch_align.
If no picts are provided, the result is nothing.
Creates a pict that combines the given picts vertically with
the first pict as topmost.
If a pict extends vertically outside its bounding box, then the
front-ot-back order of picts can matter for the combined image. The
order argument determines the order of each pict added to the
bottom of the combined image.
The picts are first made concurrent via concurrent, passing
along duration_align and epoch_align.
If no picts are provided, the result is nothing.
Creates a pict that combines the given picts by overlaying.
The order argument determines whether later picts
are placed in front of earlier picts or behind them.
The picts are first made concurrent via concurrent, passing
along duration_align and epoch_align.
If no picts are provided, the result is nothing.
Returns a
pict that draws
pict in front of or behind
on_pict at the location in
on_pict determined by
finder, where the location determined by
pinhole_finder
in
pict is matched with that location. The resulting pict’s
bounding box is the same as
on_pict’s.
The picts are first made concurrent via concurrent, passing
along duration_align and epoch_align.
> def circ = circle(~size: 16, ~fill: "lightgreen") |
|

|
|
fun connect( | ~on: on_pict :: Pict, | from :: Find, | to :: Find, | ~style: style :: ConnectStyle = #'line, | ~line: color :: ConnectMode = #'inherit, | ~line_width: width :: LineWidth = #'inherit, | ~order: order :: OverlayOrder = #'front, | ~arrow_size: arrow_size :: Real = 16, | ~arrow_solid: solid = #true, | ~arrow_hidden: hidden = #false, | ~start_angle: start_angle :: maybe(Real) = #false, | ~start_pull: start_pull :: maybe(Real) = #false, | ~end_angle: end_angle :: maybe(Real) = #false, | ~end_pull: end_pull :: maybe(Real) = #false, | ~label: label :: maybe(Pict) = #false, | ~label_dx: label_dx :: Real = 0, | ~label_dy: label_dy :: Real = 0, | ~duration: duration_align :: DurationAlignment = #'sustain, | ~epoch: epoch_align :: EpochAlignment = #'center | ) :: Pict |
|
Returns a
pict like
on_pict, but with a line added to
connect
from to
to.
|
fun table( | rows :: List.of(List), | ~horiz: horiz :: HorizAlignment || List.of(HorizAlignment) = #'left, | ~vert: vert :: VertAlignment || List.of(VertAlignment) = #'topline, | ~hsep: hsep :: Real || List.of(Real) = 32, | ~vsep: vsep :: Real || List.of(Real) = 1, | ~pad: pad :: matching((_ :: Real) | || [_ :: Real, _ :: Real] | || [_ :: Real, _ :: Real, _ :: Real, _ :: Real]) | = 0, | ~line: line_c :: maybe(ColorMode) = #false, | ~line_width: line_width :: LineWidth = #'inherit, | ~hline: hline :: maybe(ColorMode) = line_c, | ~hline_width: hline_width :: LineWidth = line_width, | ~vline: vline :: maybe(ColorMode) = line_c, | ~vline_width: vline_width :: LineWidth = line_width | ) :: Pict |
|
Creates a table
pict. For
horiz,
vert,
vsep, and
hsep, a value or final list element is
repeated as meany times as needed to cover all rows, columns, or
positions between them, and extra list elements are ignored.
> def circ = circle(~size: 16, ~fill: "lightgreen") |
> def sq = square(~size: 32, ~fill: "lightblue") |
> table([[blank(), text("Square"), text("Circle")], | [blank(), sq, circ], | [text("rolls"), blank(), text("✔")], | [text("easy to cut"), text("✔"), blank()]], | ~horiz: [#'left, #'center], | ~vert: #'top, | ~vline: "black", | ~pad: 5) |
|
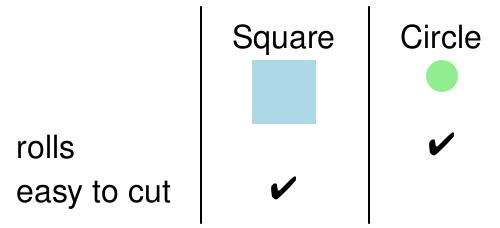
|
Creates a pict that has the total duration of the given
picts, where the resulting pict switches from one pict at the
end of its time box to the next. The result pict’s rendering before its
timebox is the same as the first pict, and its rendering after
is the same as the last pict.
If no picts are provided, the result is nothing.
Returns a list of
picts like the given
picts, except that time
boxes and epochs of each are extended to match, including the same
extent for each epoch in the time box.
If duration_align is #'pad, the time boxes are
extended as needed in the “after” direction using Pict.pad.
If duration_align is #'sustain, then
Pict.sustain is used. Note that the default for
duration_align is #'pad, but when
concurrent is called by functions like beside, the
defult is #'sustain.
The epoch_align argument determines how animations are
positioned within an extent when extents are made larger to synchronize
with concurrent, non-0 extents.
Any nothing among the picts is preserved in the
output list, but it does not otherwise particiapte in making the other
picts concurrent.
Returns a list of
picts like the given
picts, except
the time box of each is padded in the “before” direction to
sequentialize the picts.
If to_concurrent is true, then after the picts are
sequentialized, they are passed to #'concurrent. The
duration_align argument is passed along in that case.
Any nothing among the picts is preserved in the
output list, but it does not otherwise particiapte in making the other
picts sequential.
Constructs a
pict by lifting an operation on
static picts
to one on
animated picts. The
combine function is
called as needed on a list of static picts corresponding to the input
picts, and it should return a static pict.
The picts are first made concurrent via concurrent, passing
along duration_align and epoch_align.
|
fun beside.top(~sep: sep :: Real = 0, pict :: Pict, ...) :: Pict |
|
|
fun beside.topline(~sep: sep :: Real = 0, pict :: Pict, ...) :: Pict |
|
|
fun beside.center(~sep: sep :: Real = 0, pict :: Pict, ...) :: Pict |
|
|
fun beside.baseline(~sep: sep :: Real = 0, pict :: Pict, ...) :: Pict |
|
|
fun beside.bottom(~sep: sep :: Real = 0, pict :: Pict, ...) :: Pict |
Shorthands for
beside with a
~vert argument.
Shorthands for
stack with a
~horiz argument.
|
fun overlay.center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right(pict :: Pict, ...) :: Pict |
|
|
fun overlay.top(pict :: Pict, ...) :: Pict |
|
|
fun overlay.topline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.baseline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.bottom(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left_top(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left_topline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left_baseline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.left_bottom(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_top(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_topline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_baseline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_bottom(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right_top(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right_topline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right_baseline(pict :: Pict, ...) :: Pict |
|
|
fun overlay.right_bottom(pict :: Pict, ...) :: Pict |
|
|
fun overlay.top_left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.top_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.top_right(pict :: Pict, ...) :: Pict |
|
|
fun overlay.topline_left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.topline_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.center_right(pict :: Pict, ...) :: Pict |
|
|
fun overlay.baseline_left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.baseline_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.baseline_right(pict :: Pict, ...) :: Pict |
|
|
fun overlay.bottom(pict :: Pict, ...) :: Pict |
|
|
fun overlay.bottom_left(pict :: Pict, ...) :: Pict |
|
|
fun overlay.bottom_center(pict :: Pict, ...) :: Pict |
|
|
fun overlay.bottom_right(pict :: Pict, ...) :: Pict |
Shorthands for
overlay at all combinations of
~horiz
and
~vert arguments in all orders.
Options for horizontal alignment.
Options for vertical alignment.
Options for duration alignment.
Options for epoch alignment.
Options for sequential joining.
Options for overlaying.